목록Codility (5)
HEROJOON 블로그(히로블)
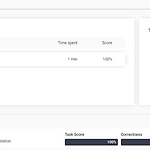
문제 Link: app.codility.com/programmers/lessons/3-time_complexity/perm_missing_elem/ 섞여있는 연속된 숫자에서 비어 있는 숫자 찾아내기 ex) [2, 4, 3, 1, 6] 연속된 숫자에서 5가 빠졌으므로 결과는 5이다. *문제에서 정확히 하나의 요소가 누락되어 있다고 했는데 누락된 숫자가 보이지 않는다면 맨 끝 숫자가 누락되었다고 볼 수 있다. 풀이 import java.util.*; public int solution(int A[]) { Arrays.sort(A); for (int i = 0; i < A.length; i++) { if (A[i] != i + 1) { return i + 1; } } return A.length + 1; //..
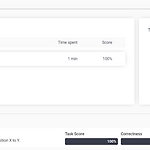
문제 Link: app.codility.com/programmers/lessons/3-time_complexity/frog_jmp/ X = 10 Y = 85 D = 30 X의 위치에서 Y위치로 이동할 때 한번에 D만큼 점프하여 이동할 수 있는데 몇 번 점프해야 Y까지 이동할 수 있을까. X의 이동된 숫자는 Y와 같거나 Y보다 커야한다. ex) 10 + 30 (한 번째 이동) + 30 (두 번째 이동) + 30 (세 번째 이동) = 100 X가 이동된 숫자 100은 Y의 85보다 크므로 이동 그만. 결과는 총 점프한 수 3 이다. 풀이 public int solution(int X, int Y, int D) { int gap = Y - X; return (gap % D) > 0 ? (gap / D) + 1..
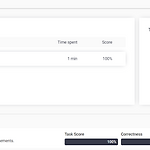
문제 Link: app.codility.com/programmers/lessons/2-arrays/odd_occurrences_in_array/ 배열에서 짝꿍이 맞는 숫자를 버리고, 홀로남은 숫자 return 하기 풀이 import java.util.*; public int solution(int[] A) { Map numMap = new HashMap(); for (int i = 0; i < A.length; i++) { if (numMap.containsKey(A[i])) { numMap.remove(A[i]); } else { numMap.put(A[i], A[i]); } } int result = 0; for (int key: numMap.keySet()) { result = key; } retu..
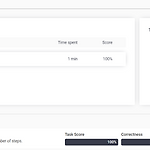
문제 Link: app.codility.com/programmers/lessons/2-arrays/cyclic_rotation/ 배열을 오른쪽으로 N번 이동하기 ex) A = [3, 8, 9, 7, 6] K = 3 가 주어졌을때 [3, 8, 9, 7, 6] -> [6, 3, 8, 9, 7] [6, 3, 8, 9, 7] -> [7, 6, 3, 8, 9] [7, 6, 3, 8, 9] -> [9, 7, 6, 3, 8] 3번 로테이션 돌렸으니까 결과는 [9, 7, 6, 3, 8] 이다. 풀이 public int[] solution(int[] A, int K) { if (A == null || A.length == 0) { return A; } int tempNum = 0; for (int i = 0; i < K..
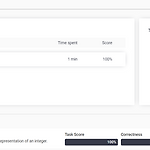
문제 Link: app.codility.com/programmers/lessons/1-iterations/binary_gap/ 가장 큰 이진 간격의 길이를 반환하라. ex) 10010001 일 경우 return 3; 만약 100000이라면 1과 1사이의 gap이 없으므로 return 0; 풀이 public int solution(int N) { String binaryStr = Integer.toBinaryString(N); char[] binaryCharArr = binaryStr.toCharArray(); int gap = 0; int maxGap = 0; for (char c: binaryCharArr) { if (c == '1') { if (maxGap < gap) { maxGap = gap; }..